Python input() function
We use the Python input() function to ask the user to enter data. We could, for example, require the user to enter a name, age, phone number, etc.
Syntax
: input(prompt)
Prompt
: (optional argument) It is the string that is a default message to user before input.
Return
: Returns the user input by converting it into a string.
Input is a function in python followed by a parenthesis. Inside the parenthesis, we specify the text that we want to print. Example, “Please enter the name”. It will prompt us to enter our name.
Example
: input(“Please enter age:”)
input("Please enter name:")
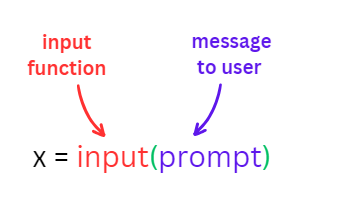
We can receive input from the user in some variables, which can be further used in different operations.
Example
: a = input(“Please enter age:”)
Prompt is an optional argument here, we can use input() without prompt as well. We will look at an example below without using a prompt.
Receiving input in a variable.
first_name = input("Please enter first name:")
print("Hello!", first_name)
Without prompt.
print("Please enter your name:")
name = input()
print("Hello!", name)
input() with typecasting
By default, the data type of value received by input is a string. We can convert it using type casting (which means converting the data type to other data types).

Here, we will first check the data type of the input using the type() function(In Python, type() function is used to check the data type of the variable.) and then, we will demonstrate an example where the user will input two numbers, and we will add them. We will save the values entered by the user in the variables. We have used the function int() for typecasting to convert string to integer for addition.
Check data type of input value.
a = input("Please enter first number : ")
b = input("Please enter second number : ")
print(type(a))
print(type(b))
input() with typecasting.
a = input("Please enter first number : ")
b = input("Please enter second number : ")
print("Sum =", int(a)+int(b))
Nested input()
Input can also be nested inside print. In this case, Python will first execute the input function and then the print function. It will first ask the user to provide the input and then execute the print statement.
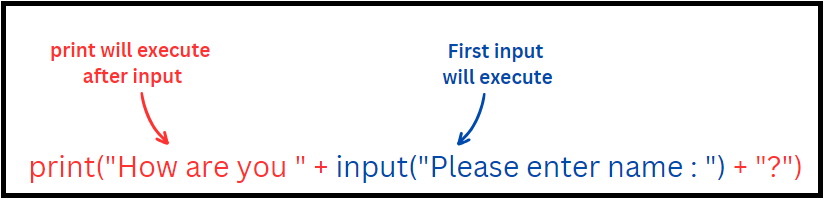
print("How are you " + input("Please enter name : ") + "?")
Multiple inputs in one line
We can ask multiple values from the user in one line. For example, in a single input, we can ask the user to enter id, name and age and save the information in the separate variables.
For this, we have to use the split function, and inside the split, we can pass the separator of our choice. For example, a comma is used as the separator in the below example.
emp_id, name, age = input("Please enter id, Full Name and age(separated by comma) : ").split(",")
print("ID: =", emp_id)
print("Name: =", name)
print("Age: =", age)
Exercise: Ask user to input two numbers and subtract them.
In this example, we will ask the user to input two numbers and subtract the second from the first one. To complete this exercise, we have to convert the numbers into integers using the int() typecasting function. This conversion will be done on the same line by wrapping the input() in the int() function.
a = int(input("Please enter first number : "))
b = int(input("Please enter second number : "))
print("Result =", a - b)
Resources
Expected Output
print("Sum =", int(input("Please enter first number : ")) + int(input("Please enter second number : ")))
Note: All this has to be done in the single line.